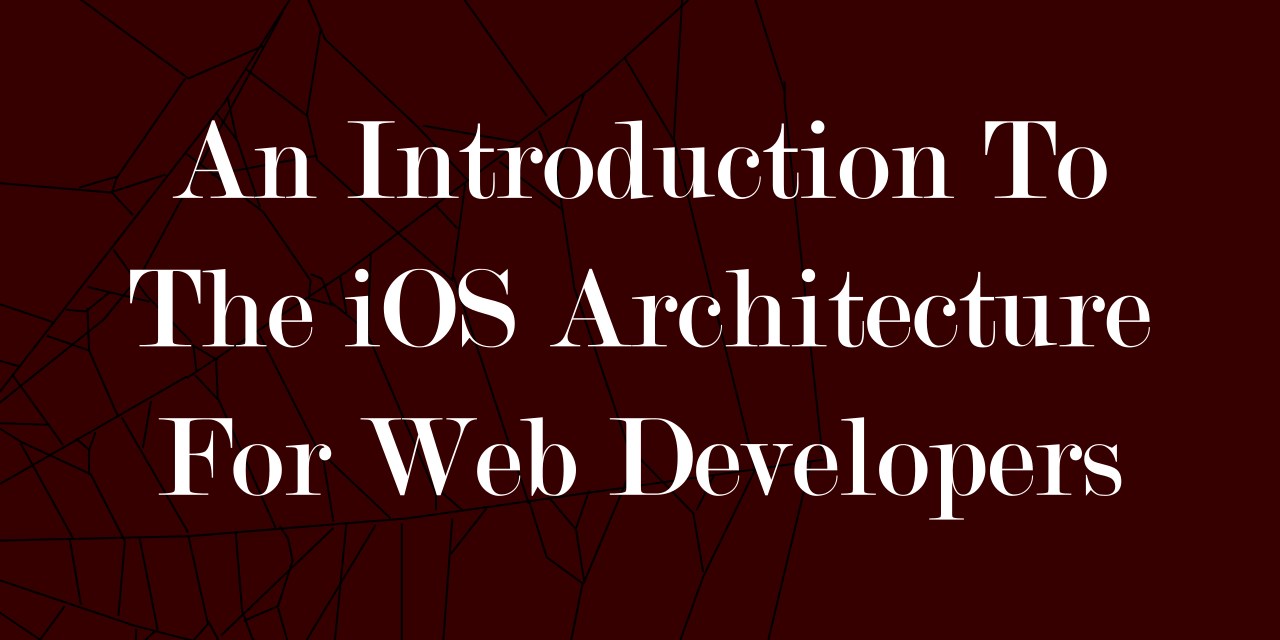
An Introduction to the IOS Architecture for Web Developers
I’ve been doing web development for a few years. I had some desktop software experience prior to that. Recently I’ve had the idea that I might like to know how to make mobile applications. I’ve played around with Android in the past and found it interesting. But at the same time I though it would be good to mix things up and do something a bit different. So I’ve started to look into iOS and iPhone development instead.
Looking at the browsers architecture
To help me get to grips with learning a new platform I like to compare and contrast it to one I already know. That way I end up having a kind of base to build off of, and it helps me internalize some of the details a bit quicker. So the question I asked myself was, how does iOS compare to the stack I’ve been using these past several years. The browser.
So before I started I wrote down everything I know about the browser and it’s architecture as a starting point for myself.
Big picture for the browser
I have to admit when I start sketching out what I know I notice a few general gaps in my own knowledge. Many specific details about how events/interactions occur were actually a little bit of a mystery to me. I happened upon a blog post By Tali Garsiel and Paul Irish on HTML5Rocks which breaks down the browser and it’s internal structures really well. A lot of the detail is too fine grained and unnecessary dense for my purposes here, but it’s actually quite valuable to know. For instance if you never understood how the layout/computed style system work (dirty bits) and how changes to the DOM can ripple out pretty dramatically then you might of questioned the point of that whole React Virtual DOM business. You might also question your own web app keeping it’s application state so tightly integrated (even stored) to the DOM in the form of data attributes/classes…etc
To save you a trip over to Tali and Paul’s article here is a quick break down:
- User Interface: This is pretty much anything except for the main rendering area of browser. Things like address bar, tabs, back and forward buttons, status bar…etc
- Browser Engine: This is a light layer that helps the user interface interact with the rendering engine…etc below it.
- Rendering Engine: This the heart of the system, in Safrai there is Webkit, in Chrome there is Blink…etc. It handles vasts amounts of functionally around parsing, understanding, consume HTML, CSS and images so that they can be present to the user.
- Networking Layer: This is a basic networking layer where everything happens; HTTP requests and such.
- JavaScript Engine: This is V8 in Chrome, SpiderMonkey in Firefox…etc. There are special bindings between the JS Engine and Rendering Engine for interacting with the DOM and CSS…etc
- UI Backend: This is a little area of the browser that handles to creation of OS specific widgets for use by the rendering engine.
So that is basically your client platform infrastructure. Obviously, a web application typically has a server side component, which could include Apache, MySQL, PHPs, Java and even now, JavaScript. I won’t go into that right now for the sake of clarity.
The type of work I do as a web developer is to:
- Write HTML/CSS/JavaScript or to describe that more formally
- Create structural elements (widget), either through HTML templating or through JavaScript (or a combination)
- Style my widget to all the needed requirements
- Make those widgets interactive through the help of the browser event system and JavaScript.
- Single Page Applications (where JavaScript controls the context of what is show).
- Networking in the form of communication calls to the server to load and save application state.
The Big Questions
So as such the question I’d have for the iOS platform is:
- What are the equivalent element in the iOS architecture that map to my stack.
- What are the languages you use there?
- What is the part of the system that handles rendering?
- What is the part of the system that handles networking?
- What is the part of the system that handles events?
- Are the events handle and exposed in a similar fashion?
- Am I able to easily bind component to certain events/interacts?
- How do I layout and style my application?
- Is there some sort of CSS for iOS?
- Is there some sort of DOM for iOS (that handles structure separately to styling/positioning)
Looking at the iOS architecture
So now that I have general sense of the architecture I typically use and questions to help me direct my exploration; I wanted to see what would be the equivalent elements in iOS that map to these browser elements.
Big picture for iOS
There are a few layer here, and none really directly translate perfectly to the browser architecture, but you get the idea. As you go down through each layer, you are getting to lower and lower levels of abstraction until you hit the Kernel (which you have almost no way to interact with). There is a lot to unpack here so lets get started.
Cocoa Touch
Cocoa Touch is high level set of APIs which developers seem to spend most of their time in. This is where a lot of the high level APIs that help developers get things done are located. There are a few key/famous APIs at this level that are really important, UIKit being an example of one. There are also mechanism exposed at this level to help developer do things like multi-tasking, laying out their application, designing the application to be responsive on multiple screen sizes, handling complex user interacts through gesture recognition, capturing application state and interacting with other applications.
In a sense the Cocoa Touch layer represent a lot of different elements within the browser rendering engine and within web application frameworks that you might use. It does not translate directly to any element within the basic browser architecture, which isn’t surprising.
Media
The Media Layer is were a lot of the more complex graphics/rendering/audio technologies relate APIs reside. If you’ve even had a passing interest in Apple announcements you might of heard of one API within this layer which is called “Metal”. Metal is supposed to be a fairly low level graphics API suitable for use by games engine developers and people who make graphically intensive applications.
The only experience I’ve had with doing low level drawing on the web was with Canvas and help from a few different JavaScript libraries. The kind of things I did there would probably happen at this level.
Other pieces in the media layer include ones centered on audio & video, to allow you to interact with the camera/video recorder and microphones of an iOS device. As far as the browser is concerned a lot of the APIs that expose the same type of functionality come from the “UI Backend” layer. You might have a high level binding in JavaScript to allow you to access the web cam (WebRTC for instance).
Core Services
Core Services is as low as most applications get. This is where you find one of the most fundamental iOS APIs, the “Foundation” API. This is a related piece of the architecture called Core Foundation which is a C based API and collection of data types which developer rarely need to go near. Foundation on the other hand is an Objective-C (and Swift) based API.
Other services at this layer are:
- SQLite
- iCloud Storage
- The Grand Central Dispatch (an API related to multi-tasking)
- The Automatic Reference Counting (ARC) system
Core OS
The Core OS is the deep dark bowels of iOS. Specifically though, this is where you would go to get fine grain control over data from the Bluetooth radio, various security related services, the Network Extension framework (for working with VPNs) and a variety of little System APIs. There is also a very interesting set of APIs for “discrete signal processing” (DSP) and other complex mathematical operations. If you wanted to get really heavy into Instragram filters this would be the place to go :P.
The Big Answers
So after a good bit of digging into the Apple Developer docs I still felt a bit mystified as to the questions I set out with, so here is a breakdown of what I’ve discovered.
What languages are used in iOS?
The native languages that I’m going to be using when developing anything in iOS seem to be:
- Swift
- Objective-C
- XML (for configuration data)
- C, but incredibly unlikely
There is also a JavaScript VM available to use a part of the overall iOS architecture. But I’m to understand that it can be quite complex to use and to interact with the rest of the system… and anyway, you’re here to learn something new, not get things done :P.
What is the part of the system that handles rendering?
Rendering is largely taken care of by the OS through a set of drawing systems called Core Graphics (formally known as Quartz). There are many layers that built on top of Core Graphics which abstract away the complex details found there. Ultimately, if all I want is a button I may never need to go too deep into the rendering engine/APIs. In the browser application world we are more concerned with rendering because it can have a real impact on application performance. Yes this is a concern to iOS developers but not in the same way as it is in the browser.
What is the part of the system that handles networking?
The primary network API is called NSURLSession, this is exposed in the Foundation framework (Core Services). If you intend on contacting third party web services or say your own server backend this is where you might want to go. However, if you were making an application primarily keeps it’s state locally on a device and you were concerned with backing that data up somewhere then you could look to using Core Data and iCloud infrastructure which are found in the Core Services instead of building out a whole crazy backend for yourself.
What is the part of the system that handles events?
Events and user interaction are baked into the UIKit and other frameworks at the Cocoa Touch level. As I mentioned previously there is a gesture recognition system found there, so I expect this to be quite straight forward to use. As for the more nuanced questions of how do I bind to certain events, I might be better off talking about those in another blog post.
Is there a DOM for iOS?
In a sense there sort of is. If by DOM do you mean a method to structurally describe you application/views you are probably talking about the UI system called Storyboard. Storyboard is a WYSIWYG UI building system which allows you to describe the different elements of your application on individual pages, and even how they connect together. So in some sense this is like writing web pages and creating hyper text references between them.
Is there some sort of CSS for iOS?
Again, there does in fact seem to be a way to achieve this. By CSS I’m probably referencing to a means by which to have fine grained control over styling/theme of my application. There is an API infrastructure called UIAppearance that seems to allow me to have a centralized location for you different application styling configuration.
There are even libraries that have emerged that build on this infrastructure and make it more abstract by allowing me to define my rules in JSON, and even something remarkably like CSS.
One of the other concerns of CSS is position. The technology that exists to handle that is probably auto layout. Auto layout is a layout constraint system built into Storyboard which seems to allow me to describe in general how I want various UI element to be position relative to the window size and other component. In effective this is how you achieve responsive applications that work on many different screen sizes.
Conclusion
I’m still at the beginning of my journey with iOS. I’ve bought a Mac Mini (which I will talk about in the future), I’m starting to get familiar with the infrastructure and architecture of the system and hoping over the coming weeks and months I’ll get to share all the ups and downs of my exploration of iOS.
References
The following links the various reference I used to write the article above. Any links I used throughout the article can be found here.
Title image
- Includes the vector image “Realistic spider web” by the Openclipart user tulvur
Articles
- “How Browsers Work: Behind the scenes of modern web browsers” By Tali Garsiel and Paul Irish
Apple Documentation
- The Cocoa Touch Documentation
- The Media Layer Documentation
- The Core Services Documentation
- The Core OS Documentation
Libraries
- UISS: the “UIKit Style Sheets” by Github user robertwijas
- nui: “Style iOS apps with a stylesheet, similar to CSS” by Github user tombenner